JavaScript Core Concepts
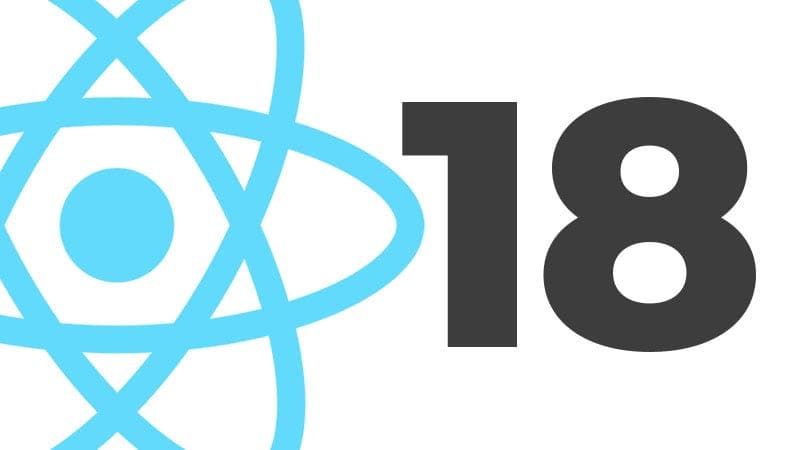
This section will introduce you to core concepts essential for learning JavaScript and TypeScript. These concepts will provide a strong foundation for your development journey.
Types and Data Structures
JavaScript is a dynamic, loosely-typed language, which means that we don't need to define variable types explicitly. However, understanding the different data types (such as strings, numbers, and booleans) and data structures (like arrays and objects) will help you write better and more efficient code.
TypeScript, on the other hand, is a strongly-typed superset of JavaScript that adds optional static typing. This means you can explicitly declare variable types, marking the difference between the two languages. You can learn more about TypeScript's type system and how it enhances JavaScript with type inference and type annotations in the TypeScript documentation.
Object-Oriented Programming
Both JavaScript and TypeScript support object-oriented programming (OOP), which is a programming paradigm that uses objects and classes to structure and organise code. You can write cleaner and more reusable code by understanding the principles of OOP, such as inheritance, encapsulation, and polymorphism.
JavaScript uses prototypes to handle inheritance and classes, while TypeScript introduces a more familiar class-based syntax for OOP, making it easier for developers with experience in other languages to transition to JavaScript development.
Key Takeways
Functions are the building blocks of any program, and understanding how to create and use them effectively is crucial for mastering JavaScript and TypeScript. Functions allow you to write reusable code and can help you manage your program's scope, which is the accessibility and lifetime of variables within your program.
JavaScript has several ways to define functions, such as function declarations, function expressions, and arrow functions. It also supports closures and higher-order functions, promoting functional programming methodologies. TypeScript extends JavaScript's function capabilities while also providing enhanced type checking and support for optional and rest parameters.
Projects and Development Environment Setup
In this section, I will discuss various aspects related to projects and development environment setup, focusing on Web Development, Development Tools, and Setting Up a TypeScript Project.
Web Development
As a developer learning JavaScript and TypeScript, building web applications is at the core of my learning journey. I focus on understanding and implementing the fundamentals of HTML, CSS, and JavaScript for frontend development, while also leveraging backend technologies such as Node.js. Creating a strong foundation in these areas enables me to build interactive and dynamic web applications that run smoothly across different browsers.
Development Tools
Having the right development tools is an essential part of my learning process. I use Visual Studio Code as my primary code editor. It offers a wide range of plugins and integrations that facilitate coding in JavaScript and TypeScript. Some of the most helpful features include syntax highlighting, autocompletion, debugging, and integrated terminal support.
Additionally, I use version control systems like Git to manage my code efficiently, as well as task runners like Grunt, Gulp, or Webpack to automate repetitive tasks such as compilation, bundling, or minification.
Setting Up a TypeScript Project
When starting a new TypeScript project, I follow these steps:
- Create a new directory for my project and navigate to it:
mkdir typescript-project && cd typescript-project
. - Initialize the project as an npm package using
npm init
and follow the prompts, which will generate apackage.json
file for managing dependencies. - Install the TypeScript compiler globally on my machine:
npm install -g typescript
, or locally for the project:npm install --save-dev typescript
. - Create a TypeScript configuration file named
tsconfig.json
usingtsc --init
, which will contain various compiler options and settings for my project. - Start coding in TypeScript using the generated configuration.
By following these steps, I ensure a consistent and efficient development workflow. A clear project structure helps me manage my code more effectively, allowing me to focus on learning and improving my JavaScript and TypeScript skills.
Advanced Features
As I continued my journey to learn JavaScript and TypeScript, I discovered some advanced features that are essential to fully harness the power of these programming languages. In this section, I'll discuss a few key advanced concepts including Interfaces and Enums, Generics and Inheritance, and Modules and Namespaces.
Interfaces and Enums
Interfaces in TypeScript enable me to define the shape of an object, ensuring that my objects adhere to a specific contract. This helps catch errors during development and improves code readability. TypeScript documentation contains great examples on how to implement interfaces.
Enums, on the other hand, allow me to define a collection of related constants, making my code more readable and self-explanatory. This is particularly useful when working with a predefined set of values, like days of the week or response statuses. I found a comprehensive introduction to Enums in this DEV Community post.
Generics and Inheritance
As I dove deeper into TypeScript, I came across Generics, which allow me to create reusable and flexible components by abstracting the type information. In this way, I can write type-safe code without compromising on flexibility. The TypeScript documentation provided helpful information on working with generics.
Inheritance is another powerful feature, especially in the context of object-oriented programming. It allows me to create classes that inherit properties and methods from other classes, leading to cleaner and more maintainable code. I found The Frontend Pro to be a valuable resource in learning more about inheritance.
Modules and Namespaces
Understanding how to work with modules and namespaces in both JavaScript and TypeScript is critical for structuring and organizing large codebases. Modules allow me to split my code into smaller, more manageable files, while namespaces help me avoid naming conflicts and provide a way to group related code. I gained valuable insights on this topic from this StackCache article.
Debugging and Best Practices
In this section, I will discuss debugging techniques and best practices for both JavaScript and TypeScript. I will cover Debugging Techniques, Design Patterns, Type Safety, and Linters as sub-sections.
Debugging Techniques
Debugging is essential to ensure the quality and correctness of our code. In JavaScript, we can make use of browser developer tools, such as the ones provided in Chrome or Firefox, to debug our code directly in the browser. For TypeScript, a very effective way to debug our code is by using Visual Studio, which offers a great set of debugging tools and features like breakpoints, variable inspection, and call stack visualization (source).
Design Patterns
Design patterns are reusable solutions to common problems encountered in software design. Applying these patterns can help improve the structure and maintainability of our code. Some of the most common design patterns in JavaScript include Module, Prototype, Singleton, and Decorator patterns. For TypeScript, we can also make use of these patterns as well as leverage additional features of the language such as interfaces, classes, and generics to create more robust and type-safe structures.
Type Safety
One of the great features of TypeScript is its support for type safety. By providing type annotations and type inference, TypeScript helps catch potential type-related errors during compile-time, making our code more robust and less error-prone. The TypeScript documentation is a great starting point for learning more about type safety and other features of the language.
Linters
Linters are invaluable tools that help identify potential issues in our code, enforce coding style, and promote best practices. They can save us time by catching errors before they become critical issues. For JavaScript, ESLint is a widely used linter that provides configurable rules to suit various coding styles and requirements. In TypeScript, we can use TSLint, which is specifically tailored for the language, although it has been deprecated and replaced by ESLint with TypeScript support (@typescript-eslint).
We help you better understand software development. Receive the latest blog posts, videos, insights and news from the frontlines of web development
Frameworks and Libraries
Let's first take a look at some popular JavaScript frameworks, namely Angular, React, and Vue.
Angular
Angular is a widely-used JavaScript framework developed by Google, designed for building dynamic web applications. It offers a comprehensive approach to application building, favoring a complete package of tools and features rather than relying on third-party libraries or plugins. Codemotion ranks it as one of the top JavaScript frameworks to learn in 2022, and I highly recommend it for those starting their journey in web development.
React and Vue
React, developed by Facebook, and Vue.js, an open-source JavaScript framework, are both known for their flexibility and component-based architecture. These two frameworks make it easier to create user interfaces for web applications, as well as handle the state management and rendering of components. Kinsta lists both React and Vue as top JavaScript libraries and frameworks for 2023, and I believe they are essential to mastering web development.
Express
Express is a minimal, unopinionated Node.js web application framework that provides a robust set of features for building web and mobile applications. Express is widely used for building server-side applications with JavaScript and TypeScript. It is essential to learn for backend development, and it's highly regarded in the JavaScript and TypeScript ecosystems. Codemotion also includes Express as a top framework for 2022.
Other Libraries
There are many other JavaScript and TypeScript libraries you can explore, depending on your interests and requirements. Some popular libraries include TensorFlow.js for machine learning, Meteor for rapid prototyping, Three.js for 3D graphics, and Dojo Toolkit for DOM manipulation. Each of these libraries offers unique features and advantages, and I encourage you to explore them as you develop your skills in JavaScript and TypeScript.
Additional Tips and Tricks
In this section, I'll share some additional tips and tricks for mastering JavaScript and TypeScript. We'll explore topics like refactoring and optimization, type narrowing and context, as well as strict mode and compile time considerations.
Refactoring and Optimization
Refactoring is the process of improving code without changing its functionality. As I progress in my JavaScript and TypeScript journey, I find that refactoring helps me maintain clean and efficient code bases. It enables me to optimize the code, improving its performance and making it easier to extend in the future.
In TypeScript, understanding types can be crucial for effective refactoring. By utilizing tools like type guards and type predicates, I can ensure that my code remains safe during the refactoring process.
Type Narrowing and Context
One powerful aspect of TypeScript is its ability to perform type narrowing. Type narrowing allows the compiler to determine a more specific type for a value, based on the context in which it is used. This can help me catch potential errors at compile time, reducing the likelihood of issues in production.
I find that using tools like the typeof
operator, type guards, and union types can help me effectively narrow the types in my code. By being aware of the context in which types are used, I can ensure that risks are minimized when working with them.
Strict Mode and Compile Time
When working with TypeScript, I often enable strict mode for my projects. Strict mode is a configuration option that enforces stricter type checking, making it less likely that I'll encounter errors during compile time. This can be especially useful when I want to maintain high-quality code for production environments.
By using strict mode, I can take advantage of TypeScript's type system to catch errors early, before they have a chance to cause issues in production. I can also leverage iterators and other advanced language features to optimize my code at compile time, ensuring that it runs efficiently in a wide range of environments.