What is React? And why use it
React is a popular open-source JavaScript library created by Facebook for building user interfaces (UI) based on components. It offers developers a powerful tool for creating web and mobile applications with reusable code snippets. React (also known as React.js or ReactJS) is maintained by Meta (formerly Facebook) and a community of individual developers and companies, making it a robust choice for app development.
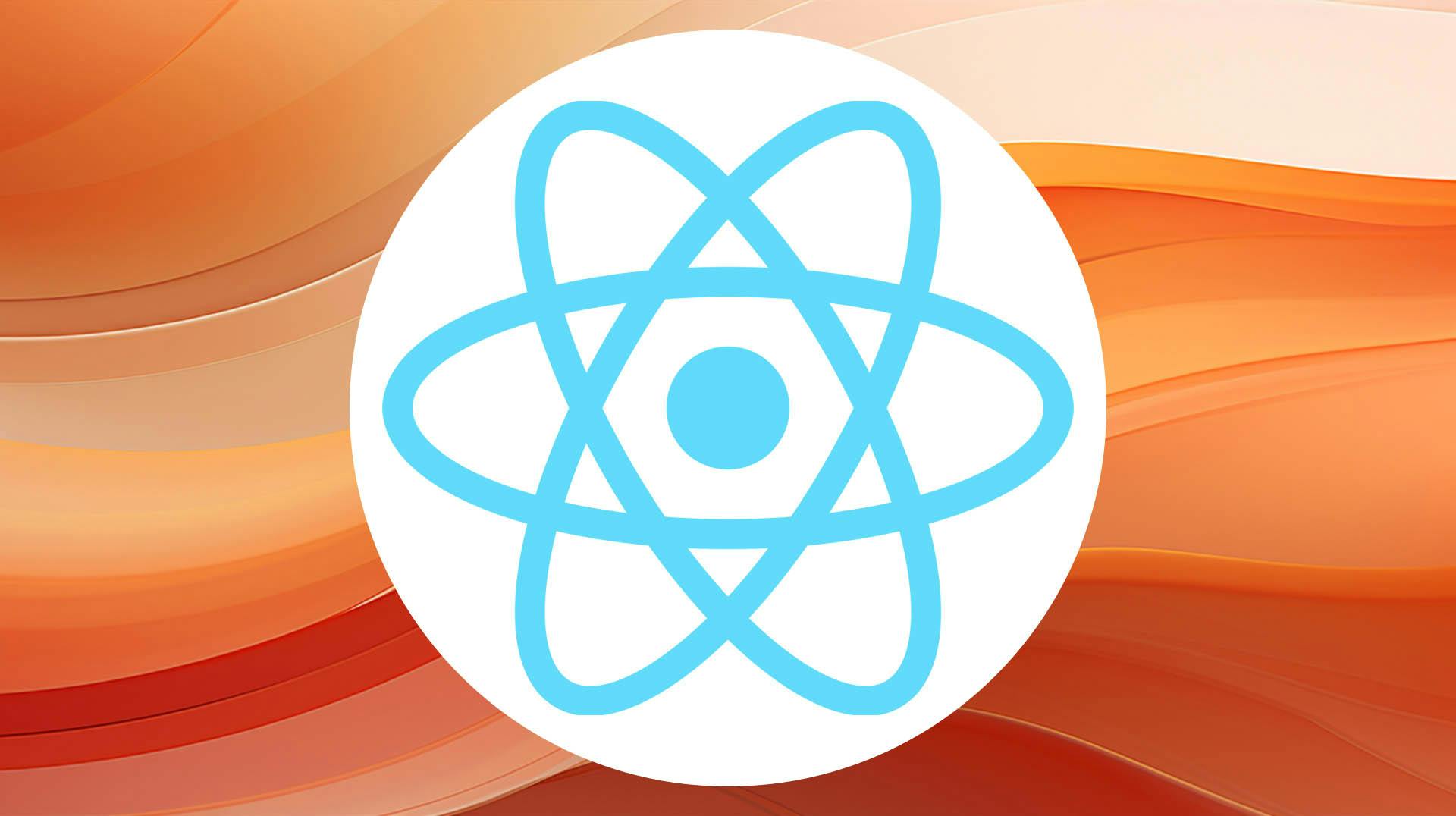
Primarily focused on frontend development, React can be integrated with other libraries to render to particular environments. For instance, React Native is used to build mobile applications for Android and iOS, while ReactDOM is used for web development. As one of the most popular JavaScript libraries, React offers a modern approach to UI building, making it essential for developers seeking to create great user experiences.
Key Takeways
- React is a popular JavaScript library for building user interfaces using components.
- It is widely used for web and mobile app development, working with other libraries like React Native and ReactDOM.
- Created by Facebook, React is maintained by Meta and a strong developer community, making it an essential tool for modern UI development.
What is React?
React has gained significant attention among developers and companies thanks to its architecture. This allows for reusable code using components. These are the building blocks of React applications. They simplify the process of building complex UIs. By breaking down the interface into smaller, independent, and reusable pieces, developers can efficiently manage and maintain code in web and mobile applications. React is not only limited to web development but also extends to mobile application development through its sister project React Native, which enables developers to build native mobile applications using React components.
How to Start with React
If you are new to React, it is advisable to have a fundamental understanding of JavaScript, HTML, CSS, and Node.js before diving into it. Once the prerequisites are covered, you can begin exploring React by following these steps.
First, make sure you have Node.js and npm (Node Package Manager) installed on your system. You can check their installation by running node -v
and npm -v
in your terminal. If you don't have them installed, download Node.js from the official website and npm will be included with it.
Next, you can use the create-react-app
package, a popular starting point for creating React applications. It conveniently sets up a new React project with a pre-configured environment and necessary tools. To set up a new React app, open your terminal and run the following command:
npx create-react-app my-react-app
Replace 'my-react-app' with your desired project name. The npx
command will install and run create-react-app
without needing to download it globally on your system. Once the process is complete, change to the project directory using cd my-react-app
and start the development server with npm start
. You will see your newly created React application running in your browser.
Why You Should Learn React
React has established itself as the leading front-end solution, consistently topping the rankings in the Stack Overflow surveys for three consecutive years. Its extensive ecosystem and large community mean there are plenty of resources for both beginners and experienced developers. This popularity and support make React an ideal choice for those seeking to excel in front-end development. Not limited to just startups, React has also been adopted by numerous major corporations, including Facebook, which developed the library. Thus, learning React increases the potential for job opportunities in various organisations, further emphasising its importance in the current job market. Beyond web development, React also enables mobile development through its React Native framework. This versatility adds more value to a developer's skill set, as they can seamlessly transition between web and mobile projects. Additionally, full-stack frameworks like NextJS are powered by React, further expanding the possibilities for developers proficient in the library. React’s popularity, supportive ecosystem, and versatility make it a valuable skill for any developer to have in their toolkit. By mastering React, developers can confidently navigate the ever-changing technological landscape and build high-quality web and mobile applications.
Features of React
What are React’s core features? JSX (JavaScript Syntax Extension): One of the essential features of React is JSX. JSX combines HTML and JavaScript, allowing developers to embed JavaScript objects within HTML elements. It makes the code easier to understand and maintain by keeping the markup and logic in the same place. Virtual DOM: React embraces the concept of the Virtual DOM, which is an in-memory representation of the web page's DOM. The Virtual DOM allows React to update only the parts of the page that need changing rather than re-rendering the entire page. This results in significant performance improvements and enhances the user experience. One-way data binding: React follows a unidirectional data flow, which means that data is passed down from parent components to child components through properties (props). This one-way data binding makes tracking and managing a component’s state easy and ensures that changes in child components do not affect their parents. Performance: React is highly efficient in managing UI updates, thanks to its use of the Virtual DOM and other optimisations. This results in faster rendering and improved application performance, making it a popular choice for web development. Conditional statements: React allows developers to use conditional statements in their code, such as ternary operators or if-else blocks. This makes it easy to render different components or display different content based on the current state of the application. Components: React's component-based architecture encourages the creation and reuse of modular components, making it easier to scale applications and maintain code quality. Components can be written by different developers, and they work seamlessly together, promoting collaboration in large projects. In summary, React offers a robust and performant solution for web development, with features like JSX, Virtual DOM, one-way data binding, and reusable components that facilitate the creation of dynamic, responsive user interfaces.
How to Code in React
Thinking in React Components
React is a JavaScript library that enables developers to build reusable UI components for single-page applications. In React, components are the basic building blocks of the user interface. Each component represents a self-contained piece of UI that can be combined with other components to create more complex and dynamic application layouts. To start coding in React, first, break down the UI into individual components and think about their hierarchy.
Using JSX in React
JSX is a syntax extension for JavaScript that allows embedding HTML-like code within JavaScript code. It enables developers to write code that combines JavaScript and HTML syntax more naturally, making it easier to work with React components. For example:
const heading = <h1>Mozilla Developer Network</h1>;
In this example, the JSX defines a simple heading component that can later be rendered within the React application. JSX, although not required, is highly recommended when working with React as it simplifies the code and improves readability.
Understanding State and Props in React
State and props are essential concepts in React for managing and passing data between components. State is an object that represents the internal data of a React component. It is mutable and can change over time, causing the component to re-render when the state changes. For instance:
class ExampleComponent extends React.Component {
constructor(props) {
super(props);
this.state = { buttonText: 'Click me' };
}
}
In this example, the ExampleComponent
has an initial state with a property called buttonText
.
Props (short for properties) pass data from one component to another. They are read-only and cannot be modified directly by the receiving component. For example:
function Greeting(props) {
return <h1>{props.message}</h1>;
}
const element = <Greeting message="Hello, world!" />;
Here, the Greeting
component receives a prop called message
, which is used to display a greeting message. By understanding state and props in React, developers can effectively manage and share data across different components in their application.
React Hooks and Functional Components
What are Hooks?
React Hooks are a feature introduced in React version 16.8 that allows functional components to access state and other React features. With React Hooks, developers can effectively do functional programming in React, making it easier to manage and reuse stateful logic. This has led to a shift in usage from class components to function components in many cases.
The useState Hook
The useState
Hook is a built-in React function that enables functional components to manage their local state. It returns an array containing the current state and a function to update that state. The basic syntax for using the useState
Hook is:
const [state, setState] = useState(initialState);
State updates are preserved across render cycles and, when called, cause the component to re-render with the new state. To update the state, simply call setState
with the new value:
setState(newState);
Here's an example of a functional component using the useState
Hook:
import React, { useState } from "react";
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
The useEffect Hook
The useEffect
Hook is another built-in React function that allows functional components to manage side effects, such as data fetching, subscriptions, or manual DOM manipulation. The useEffect
Hook runs on every render by default but can be controlled by providing an array of dependencies as a second argument.
Here is the basic syntax for using the useEffect
Hook:
useEffect(() => {
// Your effect code...
}, [dependencies]);
The effect code is executed when any of the listed dependencies change. If the array of dependencies is empty, the effect code is run only once—similar to the componentDidMount
lifecycle method in class components.
For example, to fetch data when a component mounts:
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
const response = await fetch('your-api-url');
const result = await response.json();
setData(result);
};
fetchData();
}, []);
return (
<div>
// Render fetched data...
</div>
);
}
export default FetchData;
In summary, Hooks like useState
and useEffect
empower developers to effectively manage state and side effects in functional components, leading to cleaner, more modular code and simplified component hierarchies.
Using TypeScript with React
TypeScript is a highly compatible superset of JavaScript that brings static typing to the language. It offers several advantages when used with React, such as improved code quality, developer productivity, and tooling support. React is a popular library for building user interfaces, initially developed and open-sourced by Facebook in 2013. While JavaScript is the default language used in React, TypeScript can be a valuable addition to React projects for several reasons. First of all, TypeScript ensures type safety, which helps to prevent several common issues in JavaScript, such as type coercion and undefined values. This aspect can help developers catch errors during development, resulting in fewer runtime issues and a more stable application. Additionally, the IntelliSense features provided by TypeScript make code completion and refactoring much more efficient. This aids in creating well-structured and maintainable codebases, particularly in larger teams and more complex projects. Integrating TypeScript with React is relatively straightforward. Many popular frameworks and boilerplates support TypeScript out of the box, such as Create React App, Next.js, and Gatsby. These tools make it easy to start a new React project with TypeScript and follow best practices. When working with React components, developers can leverage TypeScript interfaces to define prop types and manage component state. This approach provides better type-checking and autocompletion support when compared to PropTypes, a traditional approach to typing in React. Moreover, TypeScript works seamlessly with React hooks, enabling developers to design and implement various custom hooks with proper typing. Using TypeScript in React projects helps developers write better, more maintainable code while leveraging the full potential of the React ecosystem. By incorporating TypeScript, developers and teams can enjoy a more productive, error-free, and enjoyable development experience.
React Vs Other Frameworks
React, created by Facebook, is an open-source JavaScript library primarily focused on building user interfaces and UI components for single-page applications. Though sometimes categorised as a framework, it is technically a library. However, many developers still compare React to other well-established frameworks such as Angular and Vue due to their similar purposes in web development. Angular, developed by Google, is a comprehensive framework for building dynamic web applications. Angular follows a different philosophy. Unlike React, Angular enforces stricter and more opinionated guidelines, which can be helpful for maintaining consistency in larger projects. It uses TypeScript and offers built-in tools for handling common tasks like data binding, dependency injection, and form management. **Vue ** is another popular JavaScript framework designed for building user interfaces. It shares similarities with React and Angular but provides more flexibility in choosing their preferred style and architecture. Vue is known for its simplicity and easy learning curve, making it an attractive choice for developers of all skill levels. All three technologies, React, Angular, and Vue, are powerful and effective tools for building web applications. Their success and adoption in the industry can be attributed to several factors:
-
Performance: All these frameworks prioritise performance and responsiveness, offering efficient solutions for managing app state and rendering UI components.
-
Component-based architecture: React, Angular, and Vue all emphasise the use of components, which allows for modular and reusable code, making it simpler for developers to maintain and scale applications.
-
Strong community support: These technologies are backed by robust communities, leading to extensive documentation, third-party libraries, and diverse resources contributing to a smoother learning and development experience.
While React, Angular, and Vue cater to similar needs in the web development ecosystem, the choice between Angular, React and Vue largely depends on a project’s specific requirements and the development team’s preferences. Each technology has its own strengths and weaknesses, but all of them can deliver outstanding web applications when used effectively.
Popular Use Cases of React
React.js has gained immense popularity and is widely used in various web applications. Some of the popular use cases of React include:
- Single-page applications: React.js is well-suited for building single-page applications (SPAs) due to its component-based architecture and ability to handle a complex and interactive UI. It simplifies the development process and provides better performance than traditional multi-page applications.
- Streaming services: React.js has proven a reliable choice for developing streaming services. For example, Netflix, a leading streaming platform, uses React.
- Mobile applications: React Native, a framework based on React.js, can be used for creating cross-platform mobile applications for both Android and iOS platforms. With React Native, developers can write native mobile applications using JavaScript, significantly reducing development time while ensuring a consistent look and feel across platforms.
- E-commerce applications: React.js comes in handy for building e-commerce websites where a responsive and dynamic user interface is required. Its ability to update specific components independently means that product listings and shopping cart functionality can be updated without affecting the entire page.
- Social media applications: This was React’s first use case, since it was created at Facebook. In summary, React.js is flexible and supports a wide range of uses.